so i got a little bored and decided i needed a new project. I like the look of the zada-tech boost gauges but i didnt want to pay £95 just for a boost gauge when i reckon i can build it myself and learn some new stuff on the way
First off a couple of photo's of it on the breadboard:
Diy boost gauge by Muzza1742, on Flickr
Lcd by Muzza1742, on Flickr
I've changed the display a little, it reads boost now instead of map and the psi is displayed to 1 decimal place.
the bottom line is a re-settable peak boost display that i'm eventually gonna change over to either and oil temp or something else gauge.
Its a piece of piss to build if you've got any kind of electronics knowledge at all.
I built mine following this blog: http://blog.nsfabrication.com/2009/06/29/arduino-based-lcd-boost-gauge-with-resettable-peak-hold/
but i made some changes to his code to show the pressure in PSI instead of KPa and adjusted for 1 bar of atmospheric pressure.
Ive got the arduino mega adk instead of the uno in the parts list because the chimp in maplins got my online reservation wrong and put a £60 board in instead of the cheaper one i ordered
Parts list:
http://www.coolcomponents.co.uk/catalog/arduino-revision-p-583.html Main board £18.50
http://www.coolcomponents.co.uk/catalog/serial-enabled-16x2-black-p-472.html 16x2 Serial LCD £15.99
http://www.coolcomponents.co.uk/catalog/resistor-025w-p-339.html 10k resistor £0.10
http://www.coolcomponents.co.uk/catalog/rotary-potentiometer-linear-p-920.html 10k Pot to simulate the map sensor until i put it on the car £0.45
http://www.coolcomponents.co.uk/catalog/switch-mini-tactile-p-202.html momentary switch for the peak reset £0.28
http://uk.farnell.com/jsp/search/pr...12&s_kwcid=TC|13123|mpx4250ap||S|p|9695405829 MPX4250AP manifold absolute pressure sensor £8.74
for a total of £44.06 and a couple of hours tinkering. I've enjoyed building it and if anyone wants to have a go hopefully this post makes things a bit easier for anyone else
And now the important part: the code for the Arduino
First off a couple of photo's of it on the breadboard:
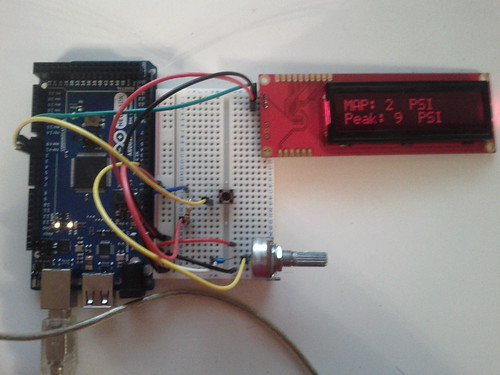
Diy boost gauge by Muzza1742, on Flickr

Lcd by Muzza1742, on Flickr
I've changed the display a little, it reads boost now instead of map and the psi is displayed to 1 decimal place.
the bottom line is a re-settable peak boost display that i'm eventually gonna change over to either and oil temp or something else gauge.
Its a piece of piss to build if you've got any kind of electronics knowledge at all.
I built mine following this blog: http://blog.nsfabrication.com/2009/06/29/arduino-based-lcd-boost-gauge-with-resettable-peak-hold/
but i made some changes to his code to show the pressure in PSI instead of KPa and adjusted for 1 bar of atmospheric pressure.
Ive got the arduino mega adk instead of the uno in the parts list because the chimp in maplins got my online reservation wrong and put a £60 board in instead of the cheaper one i ordered
Parts list:
http://www.coolcomponents.co.uk/catalog/arduino-revision-p-583.html Main board £18.50
http://www.coolcomponents.co.uk/catalog/serial-enabled-16x2-black-p-472.html 16x2 Serial LCD £15.99
http://www.coolcomponents.co.uk/catalog/resistor-025w-p-339.html 10k resistor £0.10
http://www.coolcomponents.co.uk/catalog/rotary-potentiometer-linear-p-920.html 10k Pot to simulate the map sensor until i put it on the car £0.45
http://www.coolcomponents.co.uk/catalog/switch-mini-tactile-p-202.html momentary switch for the peak reset £0.28
http://uk.farnell.com/jsp/search/pr...12&s_kwcid=TC|13123|mpx4250ap||S|p|9695405829 MPX4250AP manifold absolute pressure sensor £8.74
for a total of £44.06 and a couple of hours tinkering. I've enjoyed building it and if anyone wants to have a go hopefully this post makes things a bit easier for anyone else
And now the important part: the code for the Arduino
Code:
// NSFabrication.com Arduino boost gauge project
// Uses Freescale MPX4250AP MAP sensor on Analog port 0 and SparkFun 16×2 Serial LCD display
// Push button on D2 pulled to 5V, ground to reset peak memory
// 06-29-2009 Nick Salyer [email][email protected][/email]
int mapsen = 0; // Set MAP sensor input on Analog port 0
float boost = 0; // Set boost value to 0
float mapval = 0; // Set raw map value to 0
volatile float peakboost = 0; // Set peak memory to 0
void setup()
{
attachInterrupt (0, preset, LOW); // defines reset interrupt on D2
Serial.begin(9600); // Open serial port
Serial.write(254); // SerLCD instruction
Serial.write(01); // Clear display
Serial.write(254); // SerLCD instruction
Serial.write(128); // “Powered by:” on first line, left align
Serial.print("Seat Leon");
Serial.write(254); // SerLCD instruction
Serial.write(197); // “NSFabrication” on second line, left align
Serial.print("Cupra 1.8T");
delay (4000); // Display splash screen for 2 seconds
Serial.write(254); // SerLCD instruction
Serial.write(01); // Clear display
Serial.write(254); // SerLCD instruction
Serial.write(128); // “MAP:” at top right of display
Serial.print("BOOST");
Serial.write(254); // SerLCD instruction
Serial.write(140); // “PSI” three digits to the right of “MAP:”
Serial.print("PSI");
Serial.write(254); // SerLCD instruction
Serial.write(192); // “Peak:” at bottom right of display
Serial.print("PEAK");
Serial.write(254); // SerLCD instruction
Serial.write(204); // “PSI” left of peakboost
Serial.print("PSI");
}
void loop()
{
mapval= analogRead(mapsen); //Reads the MAP sensor raw value on analog port 0
boost = (mapval*(.00488)/(.022)*(0.145)-14.5); //Converts raw MAP value to PSI and accounts for atomospheric pressure
if (boost > peakboost) // If current boost is higher than peak, store in peak memory
{
peakboost = boost ; // Store current boost in peak memory
}
if (peakboost <= 0) // Shifts peak value 1 character to the right if < 10PSI
{
Serial.write(254); // SerLCD instruction
Serial.write(197); // Peak display after “Peak:”
Serial.print(peakboost,1); //peak to 1 decimal
}
else
{
Serial.write(254); // SerLCD instruction
Serial.write(197); // Go to position 22
Serial.write(32); // Load clear bit at position 22
Serial.write(254); // SerLCD instruction
Serial.write(198); // Go to position 22
Serial.write(32); // Load clear bit at position 23
Serial.write(254); // SerLCD instruction
Serial.write(199); // Go to position 22
Serial.write(32); // Load clear bit at position 24
Serial.write(254); // SerLCD instruction
Serial.write(200); // Go to position 22
Serial.write(32); // Load clear bit at position 25
Serial.write(254); // SerLCD instruction
Serial.write(201); // Go to position 22
Serial.write(32); // Load clear bit at position 26
Serial.write(254); // SerLCD instruction
Serial.write(202); // Go to position 22
Serial.write(32); // Load clear bit at position 26
Serial.write(254); // SerLCD instruction
Serial.write(198); // Peak display after “Peak:”
Serial.print(peakboost,1); //peak to 1 decimal
}
if (boost <= 0) //Shifts the MAP value 1 character to the right if < 10PSI
{
Serial.write(254); // SerLCD instruction
Serial.write(133); // boost after “MAP:”
Serial.print(boost,1); //boost to 1 decimal
}
else
{
Serial.write(254); // SerLCD instruction
Serial.write(133); // Go to position 6
Serial.write(32); // Load clear bit at position 4
Serial.write(254); // SerLCD instruction
Serial.write(134); // Go to position 7
Serial.write(32); // Load clear bit at position 4
Serial.write(254); // SerLCD instruction
Serial.write(135); // Go to position 8
Serial.write(32); // Load clear bit at position 4
Serial.write(254); // SerLCD instruction
Serial.write(136); // Go to position 9
Serial.write(32); // Load clear bit at position 4
Serial.write(254); // SerLCD instruction
Serial.write(137); // Go to position 10
Serial.write(32); // Load clear bit at position 4
Serial.write(254); // SerLCD instruction
Serial.write(138); // Go to position 11
Serial.write(32); // Load clear bit at position 4
Serial.write(254); // SerLCD instruction
Serial.write(134); // boost after “MAP:”
Serial.print(boost,1); // boost to one decimal
}
delay(100); // prevents LCD display from ghosting. Lower number refreshes faster, higher number has less ghosting on slow LCD’s
}
void preset() //peak reset if D2 goes low
{
peakboost = boost; //sets peakboost to current boost reading, resetting the peak memory
}